Swagger UI for NodeJS API

Swagger UI is a common way of exposing your API in a human friendly way.
Assuming you already have an API with well structured entry and exit points, but you don’t have a way to show/test them without using external tools like postman, and you want expose it to your team.
We are going to need some packages, and careful here there is one that needs to be a specific version.
Using this line on the terminal, and make sure it is on the right directory, we can get all the needed packages.
npm cors swagger-jsdoc@6.0.0 swagger-ui
swagger-jsdoc@6.0.0 is how we get the version 6 of this module. We need this because version 7 was made as an ESModule, which can’t be imported with require().
Instead of using body-parser we will use express’s own parser, first we need to import it.
const parser = require('express').json;
app.use(parser())
With cors we can get the app to accept custom headers, which we will need when we want to test it through Swagger UI.
Then with app.use('/api-docs', swaggerUI.serve, swaggerUI.setUp(specs));
we get the /api-docs route to show the swagger interface.
The object options after parsed by swaggerJSDoc() into specs creates a validated swagger specification that is then used by swaggerUI to serve a Swagger Ui page.
This also grabs @openapi and @swagger from anywhere in your code, but as a best practice we will use this in a separate file on a separate folder inside the api folder.
/**
* @openapi
* /:
* get:
* description: Welcome to swagger-jsdoc!
* responses:
* 200:
* description: Returns a mysterious string.
*/
In order to not repeat yourself you can create schemas for your objects.
/**
* @swagger
* components:
* schemas:
* YOU_OBJECT:
* type: object
* required:
* -id
* properties:
* id:
* type: string
* name:
* type: string
* address:
* type: string
*/
You can also make dividers with:
/**
* @swagger
* tags:
* name: DIVIDER
* description: DIVIDER DESCRIPTION
*/
Then we will define a method with:
/**
* @swagger
* /api/someROUTE:
* get:
* summary: Summary.
* tags: [Divider]
* responses:
* 200:
* description: Response description.
* content:
* application/json:
* schema:
* type: array
* items:
* $ref: '#/components/schemas/YOUR_OBJECT'
*/
The indentation is really important here.
The final result is a page that looks like this:
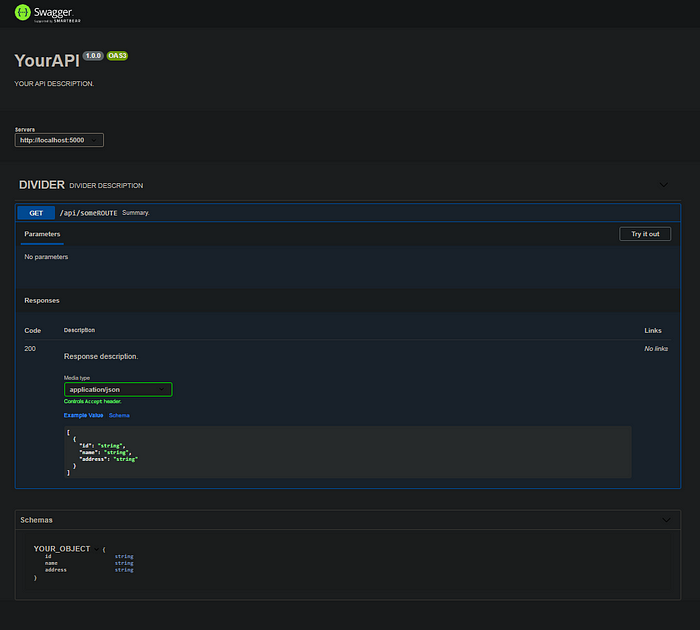